My font does not respond and it says nil
when I print it? What’s happening here?
Actually, if your UIFont
happens to be nil
, it’s maybe because you’re not calling it properly! Haha, very funny, I know, but let’s have a look at happened to me recently.
Here is what I did:
I imported "MyFont-Regular.ttf"
and "MyFont-bold.ttf"
in Xcode.
I set the constants with the font name.
let fontRegular = "MyFont-Regular" let fontBold = "MyFont-Bold"
And I set the font to that label in the view:
label.font = UIFont(name: fontRegular, size: 12.0)
and … nothing happened, well, the label was displayed but not as wanted. I expected something like label2
and label1
happened. And Label3
worked just fine btw…
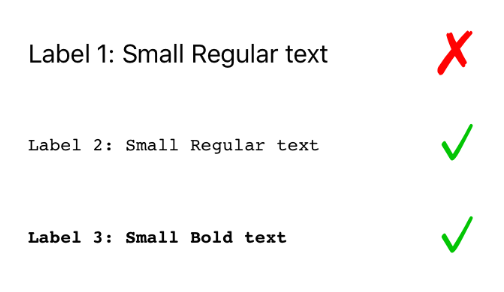
I printed the UIFont
and it failed on that line! So I double checked if my fonts were loaded correctly. And they were, so the problem was somewhere else.
Then I remembered I saw something about printing the available fonts in this article: Custom Fonts in Swift.
Those few lines can save you a lot of time and trouble:
func printMyFonts() { print("--------- Available Font names ----------") for name in UIFont.familyNames() { print(name) if let nameString = name as? String { print(UIFont.fontNamesForFamilyName(nameString)) } } }
After running the little PrintMyFonts()
function, I realized that the font I needed was called "MyFont"
by the system and not "MyFont-Regular"
. Aha!
That sounds maybe trivial, but I hope this will help you avoiding headaches and pants-over-head frustration. And remember: do not assume the font name based on the file name, even if it works sometimes ;)
Bonus: the list of default fonts supported on iOS: iOSFonts.com. It lists every font for every iOS version, for iPhone, iPad and Watch, pretty neat!